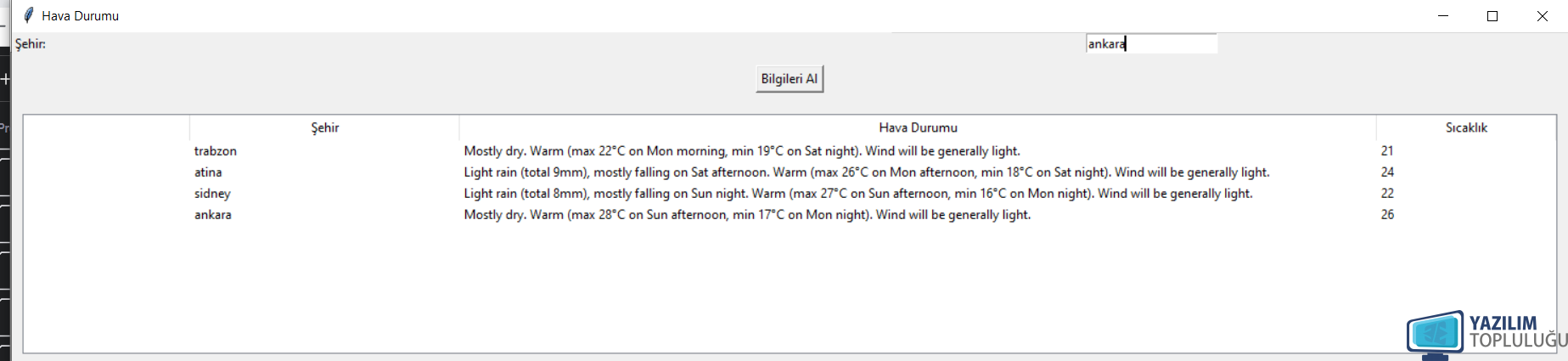
Bu kod daha önceden sorulmuştu, ama gözardı edilmesin diye yeni konuda açtım. Bugünde can sıkıntısından bir kod derledik. bu kod sayesinde "API" kullanmadan editbox nesnesine girilen şehirlere ait hava durumu bilgilerini çektik.
import requests
from bs4 import BeautifulSoup
import tkinter as tk
import tkinter.ttk as ttk
def get_weather(city):
try:
# Şehir adını içeren URL'yi oluştur
url = "https://www.weather-forecast.com/locations/{}/forecasts/latest".format(city)
# URL'ye istek gönder ve sayfayı al
response = requests.get(url)
response.raise_for_status()
# Sayfa içeriğini analiz et
soup = BeautifulSoup(response.text, "html.parser")
# Hava durumu bilgisini al
weather = soup.find(class_="b-forecast__table-description-content").get_text(strip=True)
temperature = soup.find(class_="temp").get_text(strip=True)
# Hava durumu bilgisini treeview'a ekle
treeview.insert("", "end", values=(city, weather, temperature))
except (requests.exceptions.RequestException, AttributeError):
print("Hava durumu bilgisi alınamadı.")
def on_button_click():
# Kullanıcıdan şehir adını al
city = entry.get()
# Hava durumu bilgisini al ve treeview'a ekle
get_weather(city)
root = tk.Tk()
root.title("Hava Durumu")
# Şehir giriş etiketi ve alanı
label = tk.Label(root, text="Şehir:")
label.grid(row=0, column=0, sticky="w")
entry = tk.Entry(root)
entry.grid(row=0, column=1, padx=5)
# Bilgileri al butonu
button = tk.Button(root, text="Bilgileri Al", command=on_button_click)
button.grid(row=1, column=0, columnspan=2, pady=10)
# Treeview (veri tablosu)
treeview = ttk.Treeview(root)
treeview["columns"] = ("City", "Weather", "Temperature")
treeview.heading("City", text="Şehir")
treeview.heading("Weather", text="Hava Durumu")
treeview.heading("Temperature", text="Sıcaklık")
treeview.grid(row=2, column=0, columnspan=2, padx=10, pady=10, sticky="nsew")
# Grid yöntemi ile boyut ayarları
root.grid_rowconfigure(2, weight=1)
root.grid_columnconfigure(0, weight=1)
root.grid_columnconfigure(1, weight=1)
root.mainloop()