<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
gap: 12px;
}
#bg {
background: #ddd;
width: 200px;
height: 200px;
border-radius: 100%;
}
button {
display: inline-block;
background-color: transparent;
padding: 12px;
cursor: pointer;
border-color: #ddd;
border-style: solid;
border-radius: 5px;
}
button:hover {
background: #ddd;
background-color: olivedrab;
}
</style>
</head>
<body>
<div id="bg"></div>
<button id="bg-btn">
Degis
</button>
<script defer>
const colors = [
"red",
"olivedrab",
"blue",
"fuchsia",
"aquamarine",
"orchid"]
const bg = document.getElementById("bg");
const loopByColors = async (stopIndex = 0, interval = 1000) => {
for await (const [index, color] of Object.entries(colors)) {
try {
/* her loopta promise donuyor promise resolve olduysa sonraki loopa devam ediyor
* eget reject oldu ise catch blogunda yakaliyip donguyu sonlandiriyor */
await new Promise((resolve, reject) => {
// promise icinde setTimeOut cagiriliyor
setTimeout(()=>{
// eger istenilen indexe gelinmisse
// promise reject edilip catch blogunda
// donguden cikmasi saglaniyor
if(index > stopIndex) return reject()
// degilsede arka plan rengi degistirilip promise resolve ediliyor
bg.style.background = colors[index]
resolve()
}, interval)
})
} catch (error) {
// promise reject edildiyse donguden cik
break;
}
}
}
document.getElementById("bg-btn").addEventListener("click", (e) => {
loopByColors(3, 1000) // fucshia
})
</script>
</body>
</html>
genel olarak yorum satirlari ile acikladim

edit: buda colors icine 30 tane rasgele palet icinden bir renk atiyor. son olrak bizim sectigimz bir rengide en sona atiyor.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
gap: 12px;
}
#bg {
background: #ddd;
width: 200px;
height: 200px;
border-radius: 100%;
}
button {
display: inline-block;
background-color: transparent;
padding: 12px;
cursor: pointer;
border-color: #ddd;
border-style: solid;
border-radius: 5px;
}
button:hover {
background: #ddd;
background-color: olivedrab;
}
</style>
</head>
<body>
<div id="bg"></div>
<button id="bg-btn">
Degis
</button>
<script defer>
// sirasi ile degisecek renkler
const palette = [
"red",
"olivedrab",
"blue",
"fuchsia",
"aquamarine",
"orchid"]
let colors = []
// arkaplan rengi degisecek olan div
const bg = document.getElementById("bg");
const loopByColors = async (interval = 1000) => {
for await (const [index, color] of Object.entries(colors)) {
await new Promise((resolve) => {
setTimeout(()=>{
bg.style.background = colors[index]
resolve()
}, interval)
})
}
colors = []
}
// rasgele 30 renk
const randomizeColors = (colorsLen = 30, desiredColorIndex = 0) => {
colors = []
for(let i = 0; i < colorsLen; i++) {
const randomIndex = Math.round(Math.random() * (palette.length - 1))
colors = [...colors, palette[randomIndex]]
}
colors = [...colors, palette[desiredColorIndex]]
}
document.getElementById("bg-btn").addEventListener("click", (e) => {
randomizeColors(30, 3) // fucshia
loopByColors(140)
})
</script>
</body>
</html>
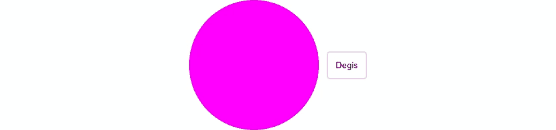