IsaacGM
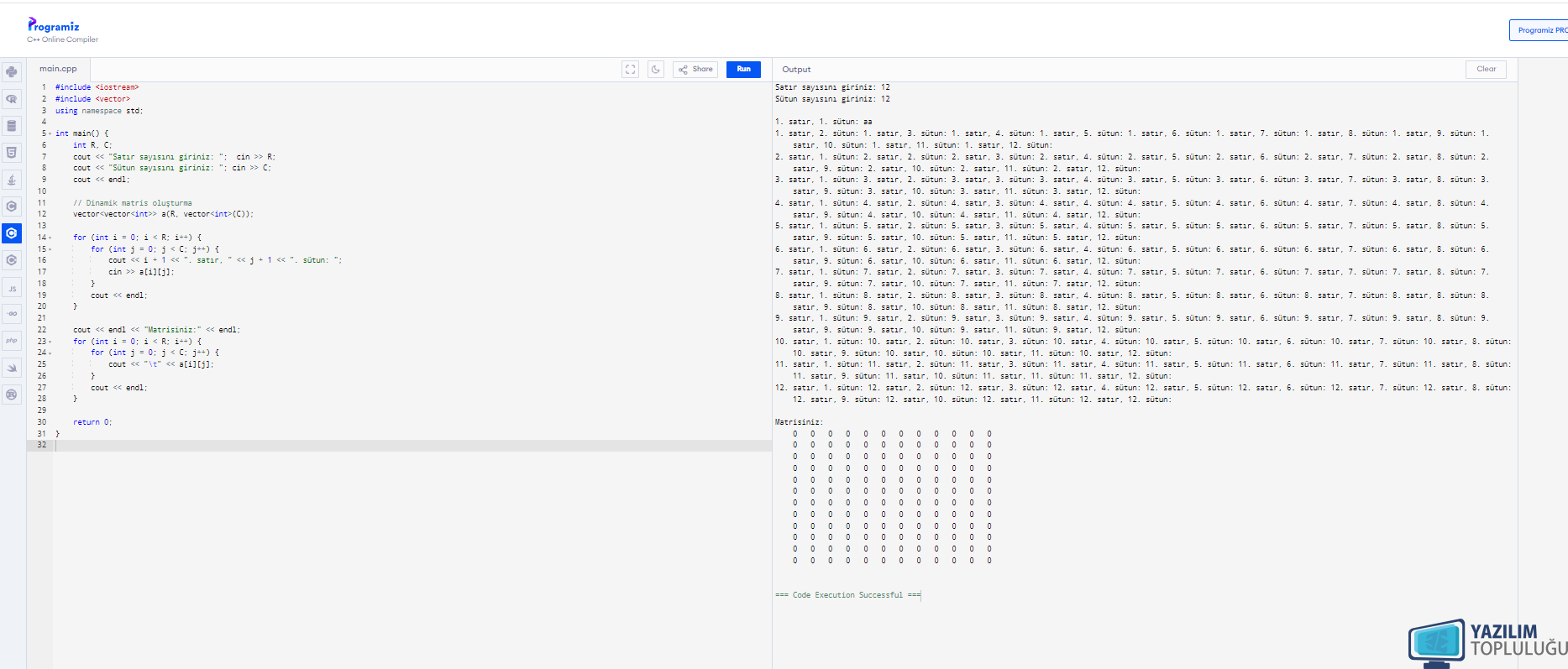
Alternative Answer;
TR:
Sorunun Analizi ve Çözüm
Yukarıdaki kodda, LINE 39'da belirtilen hata, C++ standartları nedeniyle meydana geliyor.
Hatanın Sebebi:
MS Visual Studio, varsayılan olarak C++17 veya daha üst bir standardı kullanıyor. C++17 ve üzeri standartlarda, bir dizinin boyutunun derleme zamanında sabit (constant expression) olması gerekiyor. Ancak, siz int R
ve int C
değişkenlerini kullanıcıdan alıyorsunuz, bu da dizi boyutlarının çalışma zamanında belirlendiği anlamına geliyor.
Bu durum, C99 gibi eski standartlarda desteklenirken, modern C++ standartlarında desteklenmez.
DevC++'da Çalışma Sebebi:
DevC++ genellikle GCC derleyicisini kullanır ve GCC'nin varsayılan ayarlarında, C99 genişletmeleri desteklenir. Bu nedenle, çalışma zamanı değişkeni ile dizi tanımlamak mümkün olur.
Çözüm ve Alternatifler
Çözüm 1: Dinamik Bellek Yönetimi Kullanımı (Modern C++ ile Uyumluluk)
C++'da çalışma zamanı boyutuna sahip matris için dinamik bellek yönetimi kullanabilirsiniz.
#include <iostream>
#include <vector>
using namespace std;
int main() {
int R, C;
cout << "Satır sayısını giriniz: "; cin >> R;
cout << "Sütun sayısını giriniz: "; cin >> C;
cout << endl;
// Dinamik matris oluşturma
vector<vector<int>> a(R, vector<int>(C));
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << i + 1 << ". satır, " << j + 1 << ". sütun: ";
cin >> a[i][j];
}
cout << endl;
}
cout << endl << "Matrisiniz:" << endl;
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << "\t" << a[i][j];
}
cout << endl;
}
return 0;
}
Avantajları:
- Dinamik belleği yönetmek için modern STL container'lar (ör.
std::vector
) kullanılır.
- Boyut sınırlaması yoktur; çalışma zamanı boyutları kolayca desteklenir.
- Modern C++ standartlarıyla uyumludur.
Çözüm 2: Dinamik Bellek ile new
ve delete
Kullanımı
Daha düşük seviyede, ham işaretçiler (raw pointers
) kullanarak da dinamik matris oluşturabilirsiniz:
#include <iostream>
using namespace std;
int main() {
int R, C;
cout << "Satır sayısını giriniz: "; cin >> R;
cout << "Sütun sayısını giriniz: "; cin >> C;
cout << endl;
// Dinamik dizi tanımlama
int** a = new int*[R];
for (int i = 0; i < R; i++) {
a[i] = new int[C];
}
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << i + 1 << ". satır, " << j + 1 << ". sütun: ";
cin >> a[i][j];
}
cout << endl;
}
cout << endl << "Matrisiniz:" << endl;
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << "\t" << a[i][j];
}
cout << endl;
}
// Belleği serbest bırakma
for (int i = 0; i < R; i++) {
delete[] a[i];
}
delete[] a;
return 0;
}
Avantajları:
- Daha esnek bellek yönetimi.
- Çalışma zamanı boyutları desteklenir.
- Herhangi bir standart uyumluluğu sorunu yaşanmaz.
Dezavantajları:
- Bellek sızıntılarına karşı daha dikkatli olunmalıdır.
- Daha karmaşık bir yapı.
Sorunun Nedenlerini Türkçe Açıklama
C++ Standart Farklılıkları:
- DevC++ GCC'yi kullanarak C99'u desteklediği için çalışma zamanı boyutları ile dizi oluşturmanıza izin verir.
- MS Visual Studio ise varsayılan olarak C++17 veya daha üst bir standardı kullandığından, derleme zamanında sabit olmayan boyutlarla dizi oluşturulmasına izin vermez.
Statik ve Dinamik Dizi Farkı:
- Statik dizilerde boyutlar derleme zamanında sabitlenmelidir.
- Dinamik dizilerle çalışma zamanı boyutlarına izin verilir.
Modern C++'da std::vector
Kullanımı Önerisi:
- Modern C++ standartları (C++11 ve sonrası) dinamik bellek yönetimi için
std::vector
gibi konteynerları kullanmayı önerir.
Bu çözümlerden biriyle kodunuz sorunsuz bir şekilde çalışacaktır.
EN:
Problem Analysis and Solution
In the code provided, the error on LINE 39 occurs due to differences in C++ standards.
Cause of the Error:
MS Visual Studio defaults to C++17 or later standards. In these standards, the size of an array must be a constant expression (known at compile time). However, in your code, the dimensions R
and C
are obtained from user input, meaning they are runtime values and not constant at compile time.
Why It Works in DevC++:
DevC++ typically uses the GCC compiler, which supports C99 extensions by default. C99 allows variable-length arrays (VLAs), where the size of the array can be determined at runtime. However, VLAs are not part of modern C++ standards.
Solution and Alternatives
To fix the issue and make the code compatible with modern C++ standards (C++11 and later), you can use dynamic memory allocation instead of static arrays.
Solution 1: Using std::vector
(Recommended for Modern C++)
std::vector
is a dynamic container provided by the C++ Standard Library, and it is the preferred approach for handling dynamic arrays.
#include <iostream>
#include <vector>
using namespace std;
int main() {
int R, C;
cout << "Enter the number of rows: "; cin >> R;
cout << "Enter the number of columns: "; cin >> C;
cout << endl;
// Create a dynamic 2D vector
vector<vector<int>> a(R, vector<int>(C));
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << "Enter value for row " << i + 1 << ", column " << j + 1 << ": ";
cin >> a[i][j];
}
cout << endl;
}
cout << endl << "Matrix:" << endl;
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << "\t" << a[i][j];
}
cout << endl;
}
return 0;
}
Advantages:
- Fully compatible with modern C++ standards.
- Automatically manages memory, reducing the risk of memory leaks.
- Dynamic resizing and easy to use.
Solution 2: Using new
and delete
for Dynamic Memory Allocation
If you want a lower-level solution, you can manually allocate and deallocate memory using new
and delete
.
#include <iostream>
using namespace std;
int main() {
int R, C;
cout << "Enter the number of rows: "; cin >> R;
cout << "Enter the number of columns: "; cin >> C;
cout << endl;
// Dynamically allocate memory for a 2D array
int** a = new int*[R];
for (int i = 0; i < R; i++) {
a[i] = new int[C];
}
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << "Enter value for row " << i + 1 << ", column " << j + 1 << ": ";
cin >> a[i][j];
}
cout << endl;
}
cout << endl << "Matrix:" << endl;
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++) {
cout << "\t" << a[i][j];
}
cout << endl;
}
// Free dynamically allocated memory
for (int i = 0; i < R; i++) {
delete[] a[i];
}
delete[] a;
return 0;
}
Advantages:
- Works with runtime-defined sizes.
- No reliance on C++ Standard Library containers.
Disadvantages:
- Requires manual memory management, which increases the risk of memory leaks.
- More verbose and error-prone compared to
std::vector
.
Why the Error Occurred: Step-by-Step Explanation
C++ Standard Difference:
- MS Visual Studio enforces modern C++ standards (C++17 or later), which require array sizes to be constant at compile time.
- DevC++ (using GCC) supports older standards like C99, where VLAs (Variable-Length Arrays) are allowed.
Static vs. Dynamic Arrays:
- Static arrays must have compile-time constant sizes.
- Dynamic arrays or containers like
std::vector
allow runtime-defined sizes.
Modern C++ Recommendation:
- Modern C++ prefers
std::vector
for dynamic arrays because it simplifies memory management and avoids common pitfalls like memory leaks.
Recommended Solution: Use std::vector
The use of std::vector
is the most robust and modern solution. It simplifies the code, avoids manual memory management, and ensures compatibility with C++11 and later standards.
Let me know if you need further assistance!