Başlıkta da görebileceğiniz gibi hiçbir extra kütüphane kullanmadan yalnızca json ve os kütüphanesi ile(Python dilinde default olarak bulunan kütüphanelerdir. Extra yükleme yapmanıza gerek yoktur.) basit bir veritabanı oluşturmaya çalıştım.
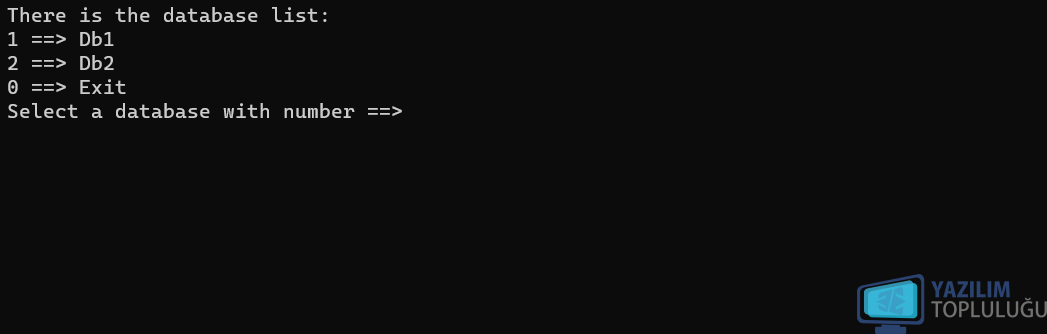
Programın olduğu klasörün alt klasörü olarak database klasörü içerisindeki json dosyalarını listeleyerek çalışmak istedğiniz veritabanını seçiyorsunuz.
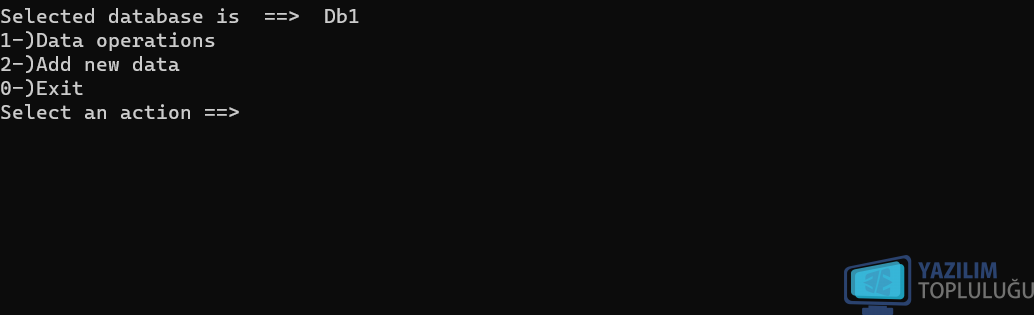
Seçtiğiniz veritabanı ile ilgili yapabileceğiniz işlemler henüz bunlar.
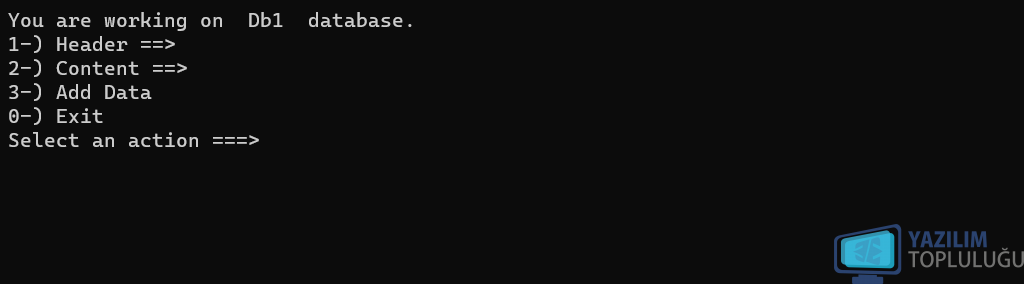
Eklenebilecek veri tipi son derece ilkel olmasına karşın kendiniz için bir not defteri olarak düşünebilirsiniz. Can sıkıntısından yazdığım bir koddu. Algoritma açısından umarım yardımcı olur.
main.py:
import os
import json
import database
def main():
os.system("cls")
def mainMenu():
while True:
while True:
try:
print("There is the database list: ")
i = 1
for item in os.listdir("database/"):
print(f"{i} ==> {item.replace('.json', '')}")
i+=1
print("0 ==> Exit")
dbSelection = int(input("Select a database with number ==> "))
if dbSelection > i-1:
os.system("cls")
print("Invalid Input")
else:
break
except:
os.system("cls")
print("Invalid Input")
if dbSelection == 0:
os.system("cls")
break
else:
os.system("cls")
print("Selected database is ==> ",os.listdir("database/")[dbSelection-1].replace(".json",""))
dataMenu(filepath= "database/"+os.listdir("database/")[dbSelection-1])
def dataMenu(filepath):
while True:
print("1-)Data operations")
print("2-)Add new data")
print("0-)Exit")
try:
selection = int(input("Select an action ==> "))
if (selection == 0):
os.system("cls")
break
elif(selection == 1):
os.system("cls")
all_data = database.findpos(filepath)
for item in all_data:
print(f'{item["id"]}-) {item["name"]}')
lastid = item["id"]
print("0-) Exit")
try:
datapos = int(input("Select data ==> "))
if datapos == 0:
os.system("cls")
continue
if(datapos > lastid or datapos < 1):
os.system("cls")
print("Invalid Input")
else:
os.system("cls")
dataOperationsMenu(filepath = filepath, datapos = datapos)
except:
os.system("cls")
print("Invalid Input")
elif(selection == 2):
os.system("cls")
dataAddMenu(filepath)
else:
os.system("cls")
print("Invalid Input")
except:
print("Invalid Input")
def dataOperationsMenu(filepath, datapos):
data = database.read(filepath,datapos)
while True:
print(f"Data Header ==> {data['name']}")
print("1-) Read Content")
print("2-) Update Content")
print("3-) Delete All Data Here")
print("0-) Exit")
try:
selection = int(input("Select an action ==> "))
if selection == 0:
os.system("cls")
break
elif selection == 1:
print(data["content"])
input("\nPress enter to continue")
os.system("cls")
elif selection == 2:
os.system("cls")
dataUpdateMenu(filepath= filepath, dataPos= datapos)
elif selection == 3:
os.system("cls")
try:
choice = input("Are you sure (y/n) ==> ")
if(choice.replace(" ", "").lower() == "y"):
os.system("cls")
delete(filepath= filepath, datapos = datapos)
print("Data Deleted Forever")
elif(choice.replace(" ", "").lower() == "n"):
os.system("cls")
print("Nothing Happened")
else:
os.system("cls")
print("Invalid Input")
except:
os.system("cls")
print("Invalid Input")
else:
os.system("cls")
print("Invalid Input")
except:
os.system("cls")
print("Invalid Input")
def dataAddMenu(filepath):
filepath = filepath.replace("database/", "")
print("You are working on ", filepath.replace(".json",""), " database.")
data = {}
data["id"] = 0
data["name"] = " "
data["content"] = " "
while True:
print("1-) Header ==> ",data["name"])
print("2-) Content ==> ", data["content"])
print("3-) Add Data")
print("0-) Exit")
try:
selection = int(input("Select an action ===> "))
if selection == 0:
os.system("cls")
break
elif( selection == 1):
header = input("Please Enter The Title of Data ===> ")
if (header.strip() == ""):
os.system("cls")
print("Invalid Input")
continue
else:
os.system("cls")
data["name"] = header
elif(selection == 2):
content = input("Please Enter The Content of Data ===> ")
if (content.strip() == ""):
os.system("cls")
print("Invalid Input")
continue
else:
os.system("cls")
data["content"] = content
elif(selection == 3):
os.system("cls")
if(data["name"].replace(" ","") == "" or data["content"].replace(" ", "") == ""):
os.system("cls")
print("Invalid Input")
continue
else:
database.add(filepath= "database/"+filepath, data= data)
data["id"] = 0
data["name"] = " "
data["content"] = " "
print("You can exit now. Data created.")
else:
os.system("cls")
print("Invalid Input")
except:
os.system("cls")
print("Invalid Input")
def dataUpdateMenu(filepath, dataPos):
data = database.read(filepath= filepath, datapos= dataPos)
while True:
print("1-) Header ==> ",data["name"])
print("2-) Content ==> ", data["content"])
print("3-) Update Data")
print("0-) Exit")
try:
selection = int(input("Select an action ===> "))
if selection == 0:
os.system("cls")
break
elif( selection == 1):
header = input("Please Enter The Title of Data ===> ")
if (header.strip() == ""):
os.system("cls")
print("Invalid Input")
continue
else:
os.system("cls")
data["name"] = header
elif(selection == 2):
content = input("Please Enter The Content of Data ===> ")
if (content.strip() == ""):
os.system("cls")
print("Invalid Input")
continue
else:
os.system("cls")
data["content"] = content
elif(selection == 3):
os.system("cls")
database.update(filepath= filepath, dataPos= dataPos, data= data)
print("Data updated successfully.")
data = database.read(filepath= filepath, datapos= dataPos)
else:
os.system("cls")
print("Invalid Input")
except:
os.system("cls")
print("Invalid Input")
def delete(filepath, datapos):
database.delete(filepath= filepath, dataPos= datapos)
mainMenu()
if __name__ == "__main__":
main()
Data işlemleri için database.py
import json
def findpos(filepath):
try:
with open(filepath,"r") as f:
data = json.load(f)
returnlist = []
for item in data:
if(item["id"] == 0):
continue
else:
returnlist.append(item)
return(returnlist)
except:
return(returnlist)
def read(filepath,datapos):
with open(filepath,"r") as f:
data = json.load(f)
for item in data:
if(item["id"] == datapos):
return(item)
def add(filepath, data):
with open(filepath,"r") as f:
temp = json.load(f)
data["id"] = temp[-1]["id"] +1
temp.append(data)
with open(filepath, "w") as f:
json.dump(temp,f,indent=3)
def update(filepath, dataPos, data):
with open(filepath,"r") as f:
temp = json.load(f)
for item in temp:
if(item["id"] == dataPos):
item["id"] = item["id"]
item["name"] = data["name"]
item["content"] = data["content"]
with open(filepath, "w") as f:
json.dump(temp,f,indent=3)
def delete(filepath, dataPos):
with open(filepath,"r") as f:
temp = json.load(f)
deletepos = 0
for item in temp:
if item["id"] == dataPos:
break
deletepos +=1
temp.pop(deletepos)
with open(filepath, "w") as f:
json.dump(temp,f,indent=3)
Bu konsol uygulamasını biraz daha geliştirmeyi düşünüyorum. Merak eden ilgisi olan olursa profilim github hesabımla bağlı.